Making Safe URLs
I need to do this often enough, I wrote a macro with some Python for it. The macro converts a url with invalid characters (such as spaces) to a safe encoded url. Importantly, the forward slashes, colons, question marks, equal signs, quotes and hashes that are necessary to pass queries and parameters are untouched by the conversion.
A common example for me is as follows. Select a URL that has bad actors in it: http://www.macdrifter.com/uploads/2011/12/$$shomer fucking?.jpg
Run the macro and the idealized URL is inserted in its place: http://www.macdrifter.com/uploads/2011/12/%24%24shomer%20fucking%3F.jpg
Here’s the Keyboard Maestro Macro:
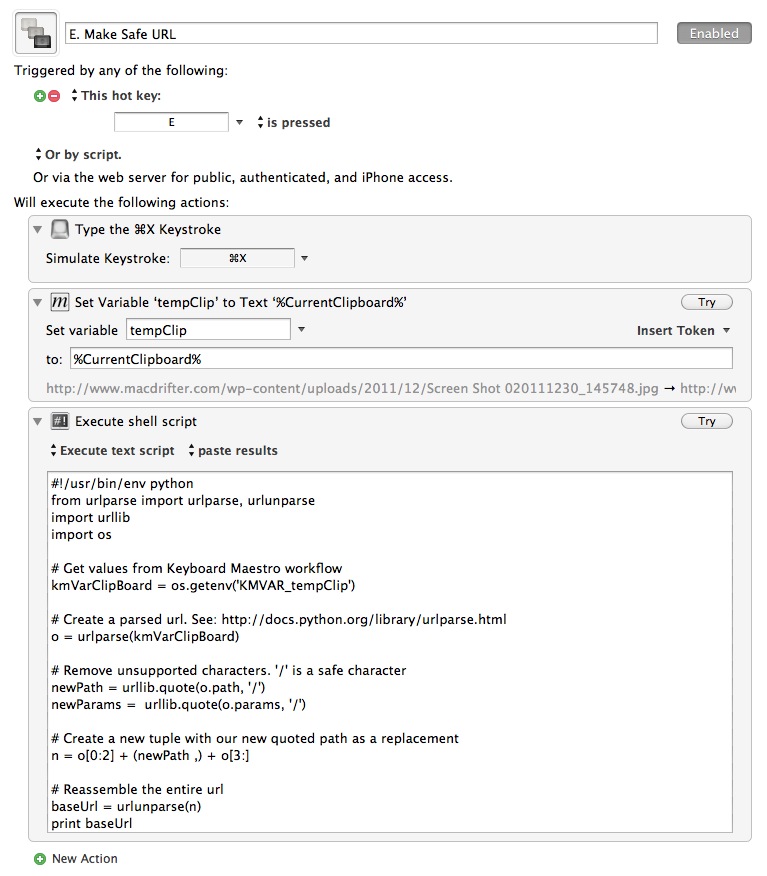
Here’s the Python script that does all of the work:
:::python
#!/usr/bin/env python
from urlparse import urlparse, urlunparse
import urllib
import os
# Get values from Keyboard Maestro workflow
kmVarClipBoard = os.getenv('KMVAR_tempClip')
# Create a parsed url. See: http://docs.python.org/library/urlparse.html
o = urlparse(kmVarClipBoard)
# Remove unsupported characters. '/' is a safe character
newPath = urllib.quote(o.path, '/')
newParams = urllib.quote(o.params, '/')
# Create a new tuple with our new quoted path as a replacement
n = o[0:2] + (newPath ,) + o[3:]
# Reassemble the entire url
baseUrl = urlunparse(n)
print baseUrl
Of course, if this is passed something that is not a url, then it will throw an exception. If that's a concern, then check one or more of the url parse values to make sure there is a domain.